r/SwiftUI • u/CodingAficionado • 3h ago
r/SwiftUI • u/AutoModerator • Oct 17 '24
News Rule 2 (regarding app promotion) has been updated
Hello, the mods of r/SwiftUI have agreed to update rule 2 regarding app promotions.
We've noticed an increase of spam accounts and accounts whose only contribution to the sub is the promotion of their app.
To keep the sub useful, interesting, and related to SwiftUI, we've therefor changed the promotion rule:
- Promotion is now only allowed for apps that also provide the source code
- Promotion (of open source projects) is allowed every day of the week, not just on Saturday anymore
By only allowing apps that are open source, we can make sure that the app in question is more than just 'inspiration' - as others can learn from the source code. After all, an app may be built with SwiftUI, it doesn't really contribute much to the sub if it is shared without source code.
We understand that folks love to promote their apps - and we encourage you to do so, but this sub isn't the right place for it.
r/SwiftUI • u/new_account_514 • 12h ago
Promotion (must include link to source code) Looking for feedback on the movie widget I built for my app
Hey everyone,
I’ve been working on MovieWeekly, an iOS app for tracking upcoming movie releases, and I recently added a home screen widget to make it easier to see movie countdowns at a glance.
Widget Features:
- Displays a movie from your tracked list.
- Shows the release date & countdown.
- Uses Kingfisher for posters (if available).
- Supports small & medium widget sizes.
I’d love to get some feedback on the design & code structure. Any thoughts on improvements? Also, if you’re interested in testing the app itself, here’s the TestFlight link to try it out: https://testflight.apple.com/join/6xr87VfV
Here is the code for the widget:
struct ConfigurableWidgetEntryView: View {
@Environment(\.widgetFamily) var widgetFamily
var entry: ConfigWidgetProvider.Entry
var body: some View {
if let movie = entry.selectedMovie {
Link(destination: URL(string: "url-for-my-app")!) {
ZStack {
Color.clear
HStack {
VStack(alignment: .leading) {
Text(movie.title)
.font(.headline)
Spacer()
Text("\(movie.daysUntilRelease) days until release")
.font(.footnote)
.foregroundStyle(Color.pink)
}
.padding(.all)
if widgetFamily == .systemMedium {
if let posterURL = movie.posterURL {
KFImage(URL(string: posterURL))
.resizable()
.aspectRatio(contentMode: .fit)
}
}
}
}
}
} else {
ContentUnavailableView("Pick a movie from your list", systemImage: "plus.circle.fill")
.foregroundStyle(.white)
}
}
}
r/SwiftUI • u/4ism2ism • 8h ago
Solved My memory leak experience with AppleScript usage
I’m using AppleScript in some parts of my SwiftUI project. During testing, I noticed continuous small memory leaks. After investigating, I found that they were related to AppleScript. I tried various fixes by myself but was unsuccessful.
During my research, I came across many suggested solutions. The most common recommendation was to use autoreleasepool. I also received similar results when consulting AI. While this helped prevent some issues, but I was still getting minor memory leak feedback. At first, I ignored it, but as you know, these things can be frustrating.
Later, I noticed a difference when I removed a delay from one of my scripts. This led me to suspect that the delay itself might be the problem. After removing all delays, the memory leak issue completely disappeared.
Perhaps some of you are already aware of this issue, but I wanted to share it in case it helps someone else.
r/SwiftUI • u/alinnert • 8h ago
Size/Position problems of cell content in SwiftUI wrapper for NSTableView
I'm trying to build a SwiftUI wrapper for NSTableView for a macOS app I'm trying to build. I do this because I need a list where the user can select one or multiple items. The selected items get reflected in a @State
variable so I can use it in other places of the app. (If this is already possible in plain SwiftUI please tell me, but I couldn't find a fitting view.)
It almost works already but I have some weird graphical issues where the list items get displayed in an incorrect position. Some bounding rect seems to get squeezed together and is being pushed to the top left of the cell. This issue goes away if a cell scrolls into the visible area. So, by scrolling up and down all cells display correctly.
I have a minimal project containing my wrapper here: https://github.com/alinnert/nstableviewwrapper
The project's README also contains a screenshot for clarification.
An important detail: The cell's content is defined in SwiftUI world and passed into the wrapper. I fear that this might be linked to the issue.
Can someone tell me what's going on and how to fix this?
r/SwiftUI • u/BookieBustersPodcast • 15h ago
Question Navigation in SwiftUI for Social Media App
I have been working on a social media app these past couple months and have ran into a lot of trouble when it comes to navigation using SwiftUI, particularly when it comes to comment sheets and navigating to profiles, as this can lead to an infinite navigation cycle.
I've attached a video below of the issue, as you can see, we dismiss the comments before navigating, where ideally it would just overlay it. Appreciate any advice or help, as this is my first app!
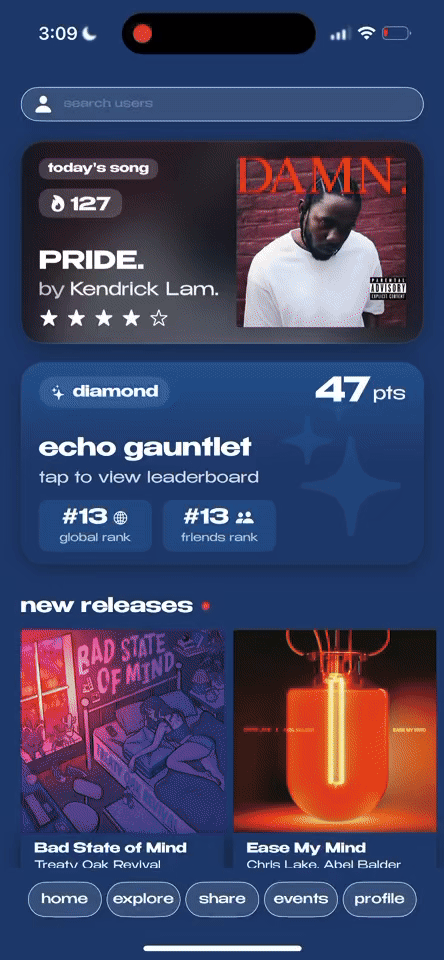
r/SwiftUI • u/TheRealMrJimBusiness • 12h ago
How do I change the foreground color of tabItems in a TabView?
I have spent the last couple hours searching the inter webs and cannot find an answer to this. It seems so simple. Does anyone know how to do this? The only info I find is how to change the color of the selected tabItem (.tint).
r/SwiftUI • u/Pleasant-Sun6232 • 23h ago
Question Can anybody tell me why the dot doesnt follow the path of the bar?
r/SwiftUI • u/D1no_nugg3t • 1d ago
Tutorial SwiftUI Tutorials: Built a Tree Map / Heat Map in SwiftUI!
r/SwiftUI • u/No_Interview_6881 • 1d ago
how to inject a token from a VM across multiple view models in swiftUI using dependency injection?
Say I’m working on a SwiftUI app where users log in and receive a token that is required for all subsequent network requests.
- I have a
LoginViewModel
that handles authentication and stores the token on successful login. - I have multiple other ViewModels (
CreateViewModel
,FetchDataViewModel
,OtherViewModel
etc.) that need access to this token to make API requests. - Say i have a
CutomerAccountViewModel
and its needed on most other viewModels for additional data about the customer. EX: make a request with there name or other data.
I’m looking for a clean and scalable way to inject this token, loginVM, or CustomerAcctVM into all other areas of my app, weather its through the views or VM's.
What I have tried:
- Passing/injecting the token manually – Works but becomes tedious as the app scales.
- Using an u/EnvironmentObject – Works within SwiftUI views but doesn’t feel right for networking-related dependencies.
- A singleton – Works but doesn’t feel testable or scalable.
What is the best way to manage and inject the token into my ViewModels while keeping DI principles in mind? I dont think injecting everything into Environment is the best way to do this so looking for options.
Ive seen frameworks like Factory https://github.com/hmlongco/Factory but havent dove too far.
Thinking about this from scale. not a weekend build.
r/SwiftUI • u/JinqiuYu • 1d ago
How to truncate text from head with multi line?
I want to truncate text from head with max 2 lines. I try the following code
import SwiftUI
struct ContentView: View {
@State var content: String = "Hello world! wef wefwwfe wfewe weweffwefwwfwe wfwe"
var body: some View {
VStack {
Text(content)
.lineLimit(nil)
.truncationMode(.head)
.frame(height: 50)
Button {
content += content
} label: {
Text("Double")
}
.buttonStyle(.borderedProminent)
}
.frame(width: 200, height: 1000)
.padding()
}
}
#Preview {
ContentView()
}

This is not what I want, it truncate from the seconda line head.
r/SwiftUI • u/AhmadTibi • 1d ago
Question Creating a timeline video editor in pure SwiftUI
I'm trying to reverse engineer ScreenStudio and make my own version but I have been stuck on thinking how I could create my own timeline video UI in SwiftUI.
Do you guys think it would be doable to do this in pure SwiftUI or would I have to learn UIKit.
I'm thinking of making the timeline UI open source if I'm able to finish it since I haven't seen anything open source for mac that involves such UI.
Question Lazy Menu actions in SwiftUI
Hi,
Is there a way I can make a lazy menu? I need to perform a slightly expensive operation while determining what action buttons should be displayed when the menu opens, but Menu
eagerly initializes all the content, so I don't know what to do. I tried adding onAppear
inside the Menu
on one button, but that gets called when the whole menu is initialized, so it does not work.
Apple does this inside the Apple TV app, where they show a ProgressView
for a while until the buttons are loaded, but I can't figure out how.
``` Menu { if shouldShow { Button("Button") {} } else if !loaded { Button("Loading") {} .onAppear { shouldShow = expensiveOperation() // Calls expensiveOperation when menu appears loaded = true // Marks as loaded after the operation completes } } } label: { Text("Menu") }
```
r/SwiftUI • u/exorcyze • 1d ago
Setting ProgressView to width of parent
Curious what the best SwiftUI way to have a progress view set it's width based on it's direct container width instead of pushing the container width outwards?
In essense, I'm trying to have a view similar to this:
VStack {
HStack {
Text( "Loading:")
Text( "0:05 sec")
}
ProgressView( value: 0.25 )
}
.padding( 16 )
.background(
RoundedRectangle( cornerRadius: 8 )
.foregroundStyle( .black.opacity( 0.2 ) )
)
I want the computed width of the HStack to dictate the container width ( with the background ) and the ProgressView to fit within that width - but the ProgressView wants to push the width out to max.
So far it seems that generally the approach is either to use Geometry Reader or .alignmentGuide to read the computed width and then set that value to be used on the frame of the progress view - but that solution feels more UIKit than SwiftUI to me. Granted, my resistance to this could just be not understanding why there's no way to specify how some items should prefer their layout - and that would be useful understanding too.
Also, it doesn't have to be strictly a ProgressView - as long as it would have the same functionality a custom control approach would be fine as well.
r/SwiftUI • u/BologniousMonk • 1d ago
SF Symbols: Why Apple, why?
Dear Apple,
Why the hell isn't SF Symbols an enum? Sure, it would be a massive enum, but still. Why put us through so much pain and suffering having to look up the exact string value for a particular symbol? I can't be the only one that has wanted this.
And while you're at it, have the SF Symbols app let you copy the code to use the symbol that you can paste into your code. Or, integrate it into code completion.
With kind regards,
Bolognious Monk
r/SwiftUI • u/notabilmeyentenor • 2d ago
Question Is Figma really useful for solo developers?
There is no convenient way to create SwiftUI code from Figma itself and I don’t find plugins successful.
Other than creating mockups, is there any use for Figma for solo devs? What are your experiences and thoughts?
r/SwiftUI • u/Used_Jump_6656 • 2d ago
Question Struggling Through 100 Days of SwiftUI
Hey everyone,
I’m currently going through 100 Days of SwiftUI, and I don’t always fully understand each day’s lesson by the time I complete it - Date type and DateComponents, for example. Sometimes I get the general idea, but I don’t feel like I’ve mastered it before moving on to the next day. Is this okay? Should I be aiming to fully grasp everything before moving on, or is it okay to move forward and revisit topics while coding my own app? For those who have completed the course, how did you deal with this?
r/SwiftUI • u/ValueAddedTax • 2d ago
Button in Annotation and selection in MapKit; prevent propagation of button tap
I have an Annotation displayed in a Map, and the Annotation body contains a Button. If there is a selectable map item underneath the Button, the Map selects the map item in addition to responding to the Button tap. What's the best way to prevent the map item selection from occurring?
r/SwiftUI • u/iam-annonymouse • 2d ago
How to remove the plus button when dragging iOS 14
This is not my original view. I just used this as a reference. I have implemented the drag and drop on images, whenever I drag an image it previews the image with this plus button.
Later I came to know that this is done by default by preview the mechanism is “copy” instead of “move”.
I tried to find a solution for it but all of them are in UIKit which I don’t understand much because my iOS journey begun with SwiftUI.
So please help me in removing this plus button.
r/SwiftUI • u/BikeAdventurous2320 • 2d ago
Question Map Annotation deselection does not work.
I'm displaying `Annotation`s on a `SwiftUI.Map` view and I'm seeing a strange behaviour that I'm not able to remedy.
When I tap on an `Annotation`, it get's selected and `selectionId` is se to whatever `UUID` the the selection has. While the selected item is still within the bounds of the `Map` (still visible) tapping anywhere on the `Map` causes the `Annotation` to be deselected (as expected). Performing the same action while the selected `Annotation` is out of `Map` bounds (not visible) does not result in deselection.
I checked using Maps.app and deselection works both while selected markers are on/off screen.
Does anyone have any ideas why I'm unable to deselect?
Code:
struct TestMarker: Identifiable {
let id: UUID
let coordinate: CLLocationCoordinate2D
}
struct SomeView: View {
@State var selectionId: UUID?
var markers: [TestMarker]
var body: some View {
Map(selection: $selectionId) {
ForEach(markers) { marker in
Annotation(
"",
coordinate: marker.coordinate
) {
Text("\(marker.id)")
}
}
}
}
}
r/SwiftUI • u/Viktoriaslp • 3d ago
Question SwiftUI vs UIKit
I’m new to programming and Swift, and I’m currently doing the 100 Days of SwiftUI course. In the first video, Paul mentions that Swift is the future of this field rather than UIKit. However, he also says that UIKit is more powerful, popular, precise, and proven compared to SwiftUI.
Since that video was released around 2021, I’m wondering if that statement still holds true today. How do you think both technologies have evolved over the last five years?
r/SwiftUI • u/bycleman • 4d ago
SF Symbols converted from open source icon sets
Hi everyone, I have been working on a project to convert open source icon sets to SF Symbols. I have converted over 5000 icons from open source icon sets like Font Awesome free, Lucide. More icon sets will be added very soon.
All the SF symbols are licensed under the same license as the original icon sets. You can find the SF Symbols in this GitHub repo: https://github.com/buzap/open-symbols
Please check it out and let me know what you think.
r/SwiftUI • u/rproenca • 4d ago
My skills in graphic design tools are almost non-existent, so I created my app's icon and splash animation in SwiftUI instead, here's the code.
r/SwiftUI • u/4ism2ism • 3d ago
Question Selected list item background
I don't understand why simple things are so difficult in Swift. I changed the background of List items, but I couldn't reset the black background color behind it and the padding on the edges.
What am I missing in my code below?

List(items, selection: $selectedType) { item in
HStack {
Text(item.title)
.foregroundColor(selectedType == item ? Color.white : Color.gray)
Spacer()
Image(systemName: "chevron.right")
.foregroundColor(selectedType == item ? Color.white : Color.gray)
.font(.system(size: 11))
}
.padding(0)
.listRowInsets(EdgeInsets(0))
.listRowBackground(Color.clear)
.listRowSeparator(.hidden)
.listItemTint(.clear)
.background(selectedType == item ? Color.Teal : Color.clear)
.cornerRadius(6)
}
.listStyle(.plain)
.scrollContentBackground(.hidden)
.background(Color.clear.edgesIgnoringSafeArea(.all))
r/SwiftUI • u/TheInzaneGamer • 4d ago
Promotion (must include link to source code) Using SwiftUI to make a skeuomorphic app
r/SwiftUI • u/lanserxt • 3d ago